Using JavaScript to Handle JWT Token Expiry
We're familiar with utilizing various authentication methods like OAuth 2.0 and API keys for REST API access. However, this article focuses on implementing JWT Token Authentication.
In this scenario, we send a resource request to the Data Endpoint using a stored JWT Token. Should the token expire or become invalid, the request fails. To address this, we refresh the token by obtaining a new one from the Login Endpoint. Subsequently, the requested data is bound to the TableGrid Control.
This tutorial exclusively employs Xano's API endpoints. Additionally, note that the JWT Token we're utilizing has an expiry after 60 seconds.
REST API Connector Configuration
- Set the Authentication to
None
- Copy the
/auth/login
endpoint from Xano and paste it in the connector’s url section and set the http method toPOST
. - Pass Raw JSON containing the
valid user credentials
to receive the JWT Token.
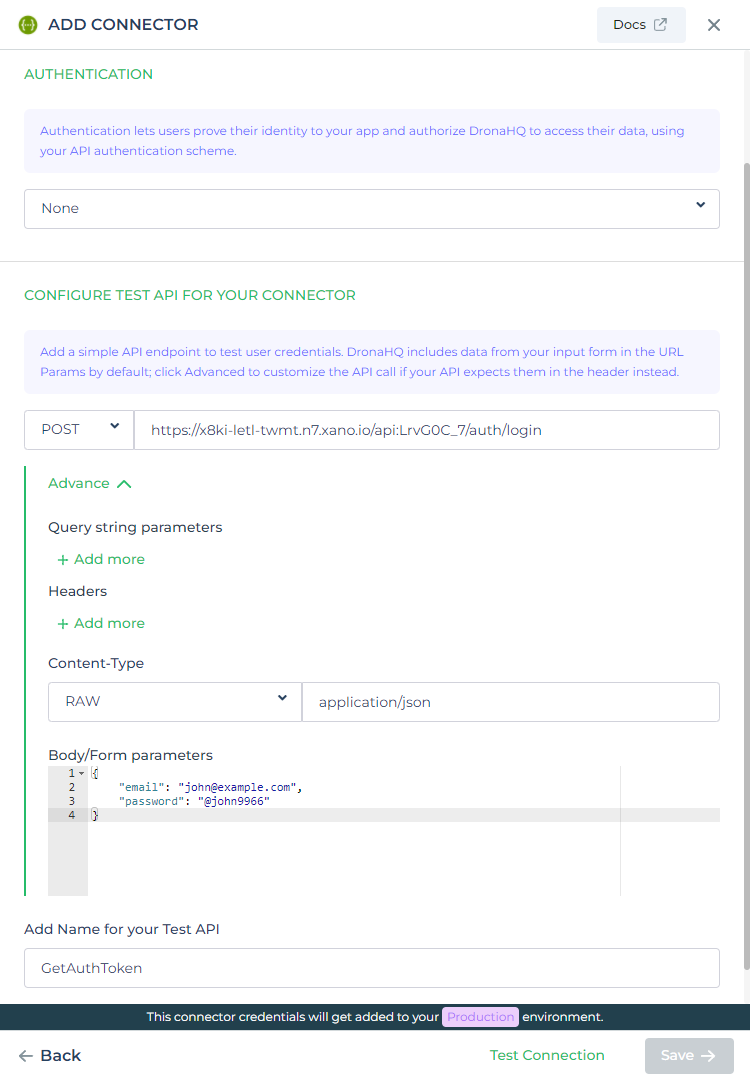
- Now add one more API to this connector, this will request data from Xano’s resource endpoint.
- Add a new header
Authorization
which will take the JWT Token as input.
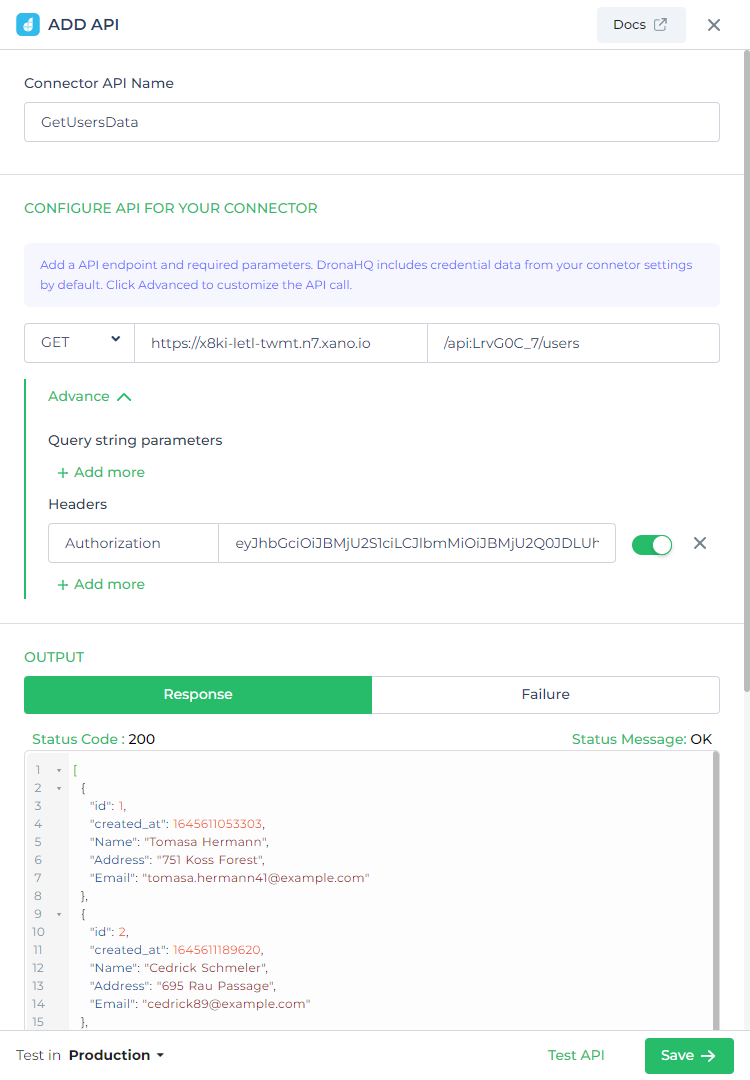
Retrieving Data from a REST API and Displaying it in a TableGrid
For this use case, we will use the Screen Open Action
to trigger data retrieval from the REST API. To display the data
accessed from the REST API, we are using the TableGrid Control.
Variable Creation: from the data query section create a new Persistent variable named
authToken
to store the JWT token. Leave the default value field blank.REST API Connector:
- Select the appropriate REST API Connector to retrieve data from the Xano endpoint.
- Within the connector's Authorization field, specify the
authToken
variable. - Introduce a new output variable named
data
to hold the response received from the REST API.
Binding Data to TableGrid:
- Upon successful retrieval by the REST API, incorporate a
Set Control Value
Action within the success branch. This action will bind the fetched data (data
variable) to the TableGrid control, enabling its display.
- Upon successful retrieval by the REST API, incorporate a
Error Handling (Expired Token):
- If the
authToken
variable holds an expired or invalid token, the REST API will respond with a 401 status code. To address this, add another REST API Connector configured to request a new JWT token from Xano's login endpoint.
- If the
New Token Storage:
- Create a variable (e.g.,
newAuthToken
) to store the newly acquired authentication token received in the API response. - Ensure you select
output.authToken
during variable assignment. This step de-structures the response and extracts only theauthToken
value.
- Create a variable (e.g.,
Updating Auth Token:
- Assign the newly acquired token (stored in
newAuthToken
) to the previously createdauthToken
variable.
- Assign the newly acquired token (stored in
Repeating Steps:
- Repeat steps 2, 3, and 4 to utilize the updated
authToken
for subsequent data retrieval.
- Repeat steps 2, 3, and 4 to utilize the updated
Verification:
- Following these steps, your App's Action Flow should resemble the provided image.
- Run the App to observe the automatic loading of API data within the TableGrid control.